Counting checkbox selections in both a Flow Formula and an LWC in Salesforce
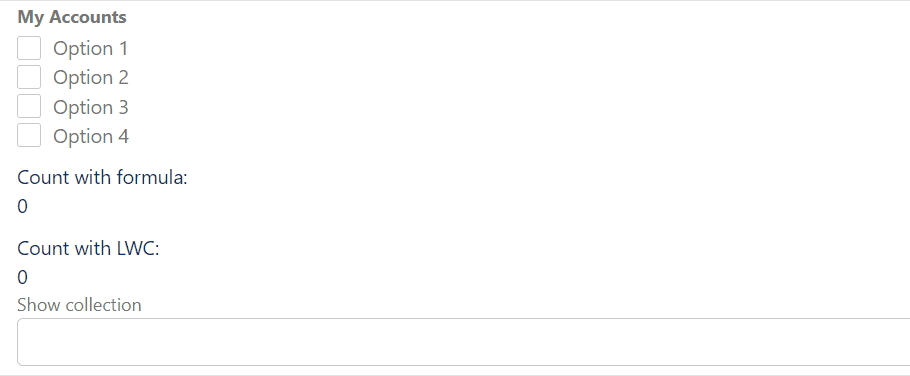
I saw a slick answer on Trailhead I wanted to highlight in this post. The original Trailhead poster asked about counting the number of selected elements in a checkbox group. A Record Choice set populates the checkbox group, and the poster's idea is to use Flow's new Reactive Flow Screens functionality to write a formula that counts the selected elements and dynamically displays the count on the same screen. Simple, right? Unfortunately, this is easier said than done!
Trailhead's Andrew Mondy then wrote the following:
This formula *SHOULD* work but there is a bug with the reactivity of checkboxes and the SUBSTITUTE function. Until SalesForce can make the SUBSTITUTE function reactive for all found instances of the "old_text" instead of only the first found instance, this solution may be impossible without custom APEX.
Assuming your checkbox component is named CheckBoxes, this formula will count the number of delimiting semi-colons in the checked choices text string, and then it adds one to it.
IF( LEN({!CheckBoxes}) = 0, 0, LEN({!CheckBoxes}) - LEN(SUBSTITUTE({!CheckBoxes}, ";", "")) + 1 )
I thought this solution was pretty neat for a few reasons:
- Instead of trying to eliminate all non ";" characters to count semicolons directly, Andrew instead takes the difference between the original string and the string when ";" characters are replaced, neat!
- This solution stays within standard Salesforce, creatively using formula functions to achieve what would have otherwise taken code.
Unfortunately, Andrew is right that at the time of writing (1/8/24), there exists a bug that prevents all instances of characters found within old_text from being used in substitution. As such, let's take a look at a side by side comparison between counting with LWC versus the Flow formula:
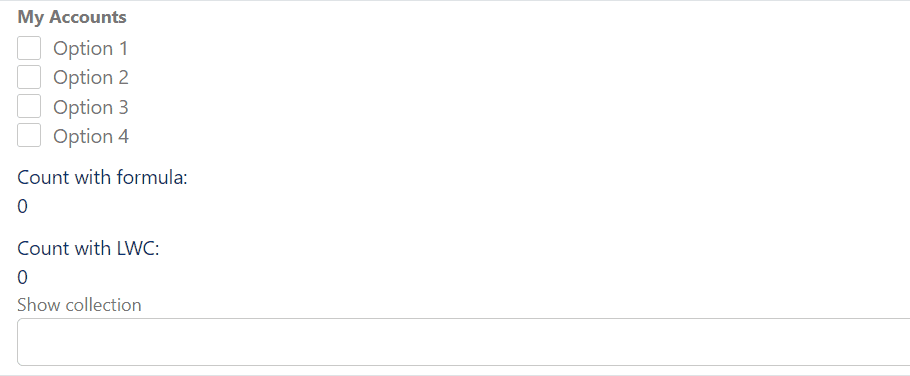
The Flow formula counts correctly at positions 0, 1, and 2. In these positions, no more than one ";" character is ever present at one time. However, after more entries are selected, the limitation with old_text substitutions prevents more countable elements.
When pivoting to LWC to count this, we have a few options at our fingertips that make counting these elements quite easy. In JS, we have the ability to use things like regex, and use split() methods (we will use split in the example). Here is how you might go about counting elements in LWC.
<template>
Count with LWC: <br>
<span>{selectedCheckboxes}</span>
</template>
countCheckboxValues.html
import { LightningElement, api } from 'lwc';
export default class CountCheckboxValues extends LightningElement {
@api selectedCheckboxes = 0;
_checkboxText;
@api
get checkboxText() {
return this._checkboxText;
}
set checkboxText(checkboxText) {
if(checkboxText){
this.selectedCheckboxes = checkboxText.split(';').length;
}
else{
this.selectedCheckboxes = 0;
}
this._checkboxText = checkboxText;
}
}
countCheckboxValues.js
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>59.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__FlowScreen</target>
</targets>
<targetConfigs>
<targetConfig targets="lightning__FlowScreen">
<property name="checkboxText" label="text" type="String" role="inputOnly" />
<property name="selectedCheckboxes" label="count" type="Integer" role="outputOnly" />
</targetConfig>
</targetConfigs>
</LightningComponentBundle>
countCheckboxValues.js-meta.xml
The JS method above simply checks if there is any content in the collection (you pass the API name of the checkbox component into the input parameter). If there is content, it will split at each ";" character. Split() returns an array with a length that we then use to count the number of selected elements in our list.
The selectedCheckboxes outputOnly annotated property can probably be removed, this was an attempt at seeing if the output of the LWC could be pushed out, used in a formula, and displayed in realtime (I was unable to get that portion to work, so I simply displayed the count within the LWC itself).
And that's all! Huge thanks to Andrew Mondy for letting me use his answer as a jumping-off point for this post!